I’m using an M1 Mac with the DesktopGL 3.8.1 template.
EDIT: I have confirmed this happens with the same example on my intel mac as well.
I am having an issue where a sprite drawn at the mouse position lags behind immediately after a left click if the mouse is moving when the left click occurs. This does not happen with other mouse button clicks.
- I thought at first that my game was dropping frames, but confirmed that to be false.
- Then, I thought it was a delay from by BT mouse, so I tried a USB wireless mouse (no dice).
- I tried a wired mouse (same deal)
- I tried on my MacBook screen instead of on a monitor (same issue with trackpad or mouse)
- Then, I tried windows (issue doesn’t appear there)
- Finally, I decided to make the most minimal example to see if anything with my game was causing it (still the same issue).
So, below I have the code for this example.
My best guess right now is that somewhere something is trying to detect a mouse drag gesture since the click and movement are happening at the same time, and somehow during that detection interval the mouse position doesn’t get updated.
Would love to figure out what’s happening here!
Behavior without clicking:
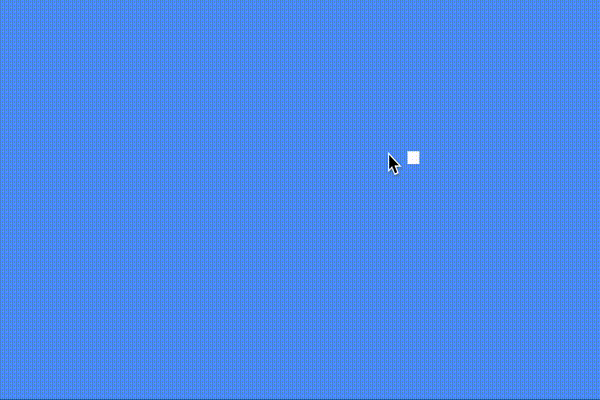
Behavior with clicking (I also turned on mouse clicks in the screen recording):
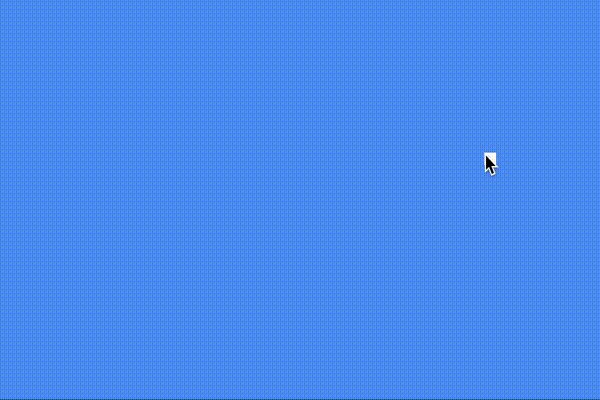
Code:
using Microsoft.Xna.Framework;
using Microsoft.Xna.Framework.Graphics;
using Microsoft.Xna.Framework.Input;
namespace MouseButtonLagTest;
public class Game1 : Game
{
private GraphicsDeviceManager _graphics;
private SpriteBatch _spriteBatch;
private Texture2D whiteTexture;
private MouseState currentMouseState;
public Game1()
{
_graphics = new GraphicsDeviceManager(this);
Content.RootDirectory = "Content";
IsMouseVisible = true;
}
protected override void Initialize()
{
base.Initialize();
}
protected override void LoadContent()
{
_spriteBatch = new SpriteBatch(GraphicsDevice);
whiteTexture = new Texture2D(GraphicsDevice, 1, 1);
whiteTexture.SetData(new[] { Color.White });
}
protected override void Update(GameTime gameTime)
{
currentMouseState = Mouse.GetState();
base.Update(gameTime);
}
protected override void Draw(GameTime gameTime)
{
GraphicsDevice.Clear(Color.CornflowerBlue);
_spriteBatch.Begin();
_spriteBatch.Draw(
texture: whiteTexture,
position: currentMouseState.Position.ToVector2(),
sourceRectangle: null,
color: currentMouseState.LeftButton == ButtonState.Pressed ? Color.Red : Color.White,
rotation: 0f,
origin: Vector2.Zero,
scale: 10 * Vector2.One,
effects: SpriteEffects.None,
layerDepth: 1f
);
_spriteBatch.End();
base.Draw(gameTime);
}
}
This is odd, especially because you can reproduce it with a minimal example (I see nothing wrong with your code) and because you can’t reproduce it on Windows. To confirm, this only happens in a MonoGame project and not elsewhere, like other applications or just your desktop, right?
It might be a good idea to bring this to the attention to the devs, there might be an issue on your platform specifically. I’m not sure the best way to do that… but maybe posting an issue on github could help?
can you try to use Vector2 in Update to get the mouse position and use it to draw instead of caching the mouse.GetState()?
1 Like
Are you using the trackpad or a physical mouse?
Are you using the trackpad or a physical mouse?
Issue happens with either one
To confirm, this only happens in a MonoGame project and not elsewhere, like other applications or just your desktop, right?
Yeah I haven’t found another app that has this behavior…
but maybe posting an issue on github could help?
Good point. I’ll do some more testing and post something there. Thanks!
can you try to use Vector2 in Update to get the mouse position and use it to draw instead of caching the mouse.GetState()?
Just tried this and had the same result (thanks for the idea though).
To make sure I understood you right, I did this in the update:
mousePosition = Mouse.GetState().Position.ToVector2();
And then passed position: mousePosition
into the SpriteBatch.Draw function.
Your code is fine. Are you using a Bluetooth mouse on your Mac? If so, try a wired mouse and see what happens. This is just to test if your Bluetooth is having problems due to interference.
try a wired mouse and see what happens. This is just to test if your Bluetooth is having problems due to interference.
I’ve tried it on a BT mouse, a usb wireless mouse (dongle), and a usb wired mouse. All the same 
I wonder if anyone else with a mac can replicate this?
I ran your code on an M1 Mac Mini with both a blue tooth Magic Mouse and a wired USB mouse and everything was fine.
I’m really confused then because this happened on two different MacBooks. One M1 and one intel…
Thanks much for trying that though! I’ll keep thinking… Something in the carpet here maybe
I have an update!
If I apply both of these settings, it goes away:
GraphicsDeviceManager.SynchronizeWithVerticalRetrace = false
Game.IsFixedTimeStep = false
I was doing this to improve FPS elsewhere, but applying it to this example solves the issue. Still unclear why, but nice!
PS: Here are the results of all four combos of these settings:
-
fixed timestep OFF, vsync OFF: Works great, no noticeable lag
-
fixed timestep OFF, vsync ON: Small lag noticeable
-
fixed timestep ON, vsync OFF: Significant lag
-
fixed timestep ON, vsync ON: Significant lag
And here’s the updated game init:
public Game1()
{
_graphics = new GraphicsDeviceManager(this);
_graphics.SynchronizeWithVerticalRetrace = false;
_graphics.ApplyChanges();
Content.RootDirectory = "Content";
IsMouseVisible = true;
IsFixedTimeStep = false;
}
2 Likes
I’m glad you figured it out! With that said…
I’m wondering if this is still worth an issue logged. Now that you’ve identified this, it might not be a bad idea to go run through your other tests you did above on other machines/platforms to see if you can reproduce the issue there.
If you can’t and it’s still MacOS only for you, perhaps @David_Lent can give it another go with this new found information? If it does turn out this is a MacOS only issue, logging a bug might help the dev team explore this and see if there needs to be a fix 
Definitely worth! Issue posted here: Lag on left mouse button click on macOS · Issue #8011 · MonoGame/MonoGame · GitHub
I tried all cases above on an intel machine and got the same result.
1 Like
I have the same issue on other applications, how to solve it out of monogame ?