if its just for simple testing you can just use spritebatch to draw lines using regular old draw.
public static void DrawBasicLine(Vector2 s, Vector2 e, int thickness, Color linecolor)
{
spriteBatch.Draw(dot, new Rectangle((int)s.X, (int)s.Y, thickness, (int)Vector2.Distance(e, s)), new Rectangle(0, 0, 1, 1), linecolor, (float)Atan2Xna(e.X - s.X, e.Y - s.Y), Vector2.Zero, SpriteEffects.None, 0);
}
public static float Atan2Xna(float difx, float dify)
{
if (SpriteBatchAtan2)
return (float)System.Math.Atan2(difx, dify) * -1f;
else
return (float)System.Math.Atan2(difx, dify);
}
A curve is just a series of lines if you need to draw a curve faster dynamically.
You’ll probably need to do so on a shader or something that’s a pretty complicated topic.
Statically you can use the line strategy and buffer the lines into a vertice buffer.
Varying the time below will give you points on the curve to use to form line segments.
You could put this onto a shader rendering a quad around the drawing area.
public Vector3 CalculateBspline(Vector4 a0, Vector4 a1, Vector4 a2, Vector4 a3, float time)
{
var a4 = (a1 - a0) * time + a0;
var a5 = (a2 - a1) * time + a1;
var a6 = (a3 - a2) * time + a2;
var a7 = (a5 - a4) * time + a4;
var a8 = (a6 - a5) * time + a5;
var a9 = (a8 - a7) * time + a7;
solved[0] = a0;
solved[1] = a1;
solved[2] = a2;
solved[3] = a3;
solved[4] = a4;
solved[5] = a5;
solved[6] = a6;
solved[7] = a7;
solved[8] = a8;
solved[9] = a9;
return ToVector3(a9);
}
This is a incomplete sort of test project i started not sure were i was going with it really but game2 i believe will draw a simple subdivision bezier b-spline.
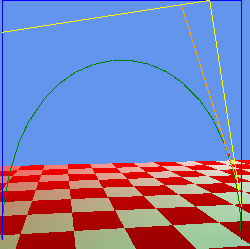