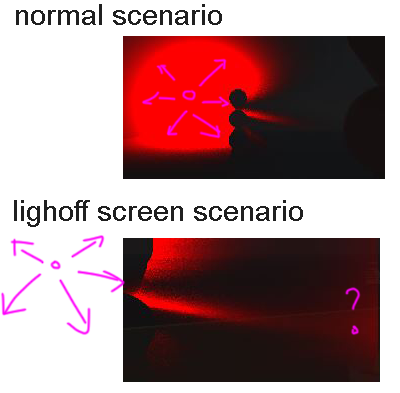
I got it to work by modified the code as below, but Iâm having a light leak issue when my light origin is offscreen or behind the camera.
RayMarching Function :
float4 RayMarch(float2 screenPos, float3 rayStart, float3 rayDir, float rayLength, float C2R, float3 cullRayStart, float3 cullRayEnd)
{
float offset = (frac(sin(dot(screenPos /**(frac(_iTime) + 1)*/, float2(15.8989f, 76.132f))) * 46336.23745f));
int _SampleCount = 24;
int stepCount = _SampleCount;
float stepSize = rayLength / stepCount;
float3 step = rayDir * stepSize;
float3 currentPosition = rayStart + step * offset;
float4 vlight = 0;
float cosAngle;
float3 cullRayDir = cullRayEnd - cullRayStart;
// we donât know about density between camera and lightâs volume, assume 0.5
float extinction = length(_cCamPosWS - currentPosition) * _volumetricData.y * 0.5;
[unroll]
for (int i = 0; i < stepCount; ++i)
{
float4 rayPosition = float4(float3(cullRayStart + ((i + offset)/(_SampleCount)) * cullRayDir), 1);
float atten = GetLightAttenuation(currentPosition);
float density = 1.0;//GetDensity(currentPosition);
float scattering = _volumetricData.x * stepSize * density;
extinction += _volumetricData.y * stepSize * density; // +scattering;
float4 light = atten * scattering * exp(-extinction);
if (length(rayPosition.xyz) < C2R - 0.99)
vlight += light;
currentPosition += step;
}
return vlight;
}
PointLight Function :
float3 posVS = max(0.01, lineardepth) * cameraDirVS;
float3 wpos = mul(float4(positionVS.xyz, 1), _Mat_InvV).xyz;
float3 rayStart = _cCamPosWS;
float3 rayEnd = wpos.xyz;
//float3 rayEnd = wpos;
float3 rayDir = (rayEnd - rayStart);
float rayLength = length(rayDir);
rayDir /= rayLength;
float projectedDepth = (lineardepth) / dot(_CameraForward, rayDir);
float L2C = length(_lightPosition);
float C2R = distance(float3(0, 0, 0), positionVS);
float3 cullRayStart = normalize(i.positionVS.xyz) * (L2C - _lightRadius);
float3 cullRayEnd = normalize(i.positionVS.xyz) * (L2C + _lightRadius);
float3 lightToCamera = _cCamPosWS - _LightPosWS.xyz;
//lightToCamera -= lightToCamera;
float b = dot(rayDir, lightToCamera);
float c = dot(lightToCamera, lightToCamera) - (_lightRadius * _lightRadius);
float d = sqrt((b * b) - c);
float start = -b - d;
float end = -b + d;
end = max(end, projectedDepth);
rayStart = rayStart + rayDir * start;
rayLength = end - start;
o.Volume = RayMarch(i.screenPos.xy, rayStart, rayDir, rayLength, C2R, cullRayStart, cullRayEnd);