There is a bit of work to add a player and collisions.
Take a look at monogame.extended.collisions.
This is what I am doing (in my main project - not the TiledExample)
- create a Tile class which implements ICollisionActor interface
- create a Player class which implements ICollisionActor interface
- create a CollisionComponent class which handles the collisions
- create an array of Tile which corresponds to the tiles in the map
- Add all the blocking tiles and the player to the CollisionComponent.
Follow the docs above to setup the OnCollision method in the Player classes.
The Tile OnCollision doesnāt need to do anything.
do you want to share that example too if finished?
i have no idea how to do it 
Canāt it be made like the video i showed but with code from monogame.extended to read the ācollisionsā layer?
Because your way seems much more work/difficult.
thx
Lisa
Can you tell me how to read a layer of a Tiled map with name ācollisionsā by using monogame.extended?
Maybe I can replace the code of the video then by this monogame.extended code.
The video is using TiledSharp which is no longer supported (also I found it unwatchable unless I muted the horrendous music
)
Iām not using a separate layer for collisions - I added a custom attribute to each tile (in Tiled) to indicate whether it blocks movement.
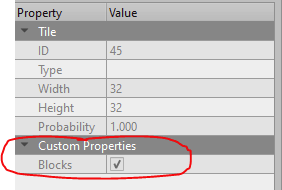
You need to read up on the monogame.extended.collisions docs and understand them before you can proceed.
I literally followed the example code on their page.
Take bite sized pieces and work on each one before trying the next ābiteā:
- get your Tiled map displayed on the screen
- get your player character displaying on the screen
- allow the player to be moved around using the keys
- Add in collision support by following the monogame.extended.collisions example.
This code sets up the array of Tile objects - one for each tile in the map.
It looks up the tile at Layer[0] to see if it blocks and if it does it adds it to the collisions handler.
// member variables
private TiledMap _tileMap;
private TiledMapRenderer _tiledMapRenderer;
private OrthographicCamera _camera;
private Vector2 _cameraPosition;
private CollisionComponent _collisionHandler;
...
_collisionHandler = new CollisionComponent(new RectangleF(0, 0, 800, 600));
...
public void InitialiseTiles()
{
_tileMap = GraphicsManager.Instance.GetMap();
_tiledMapRenderer = new TiledMapRenderer(GraphicsManager.Instance.GetGraphicsDevice(), _tileMap);
var viewportadapter = new BoxingViewportAdapter(GraphicsManager.Instance.GetWindow(), GraphicsManager.Instance.GetGraphicsDevice(), 800, 600);
_camera = new OrthographicCamera(viewportadapter);
_cameraPosition = new Vector2(512, 384);
_tiles = new LevelTile[_tileMap.Width, _tileMap.Height];
for (int i = 0; i < _tileMap.Width; i++)
{
for (int j = 0; j < _tileMap.Height; j++)
{
int tileType = -1;
var tile = _tileMap.TileLayers[0].GetTile((ushort)i, (ushort)j);
var tileSet = _tileMap.GetTilesetByTileGlobalIdentifier(tile.GlobalIdentifier);
if (tileSet == null) continue;
tileType = GetTileId(_tileMap, tileSet, tile.GlobalIdentifier);
if (tileType < 0) continue;
_tiles[i, j] = new LevelTile(i, j, tile.GlobalIdentifier, 0);
if (tileSet.Tiles[tileType].Properties.ContainsKey("Blocks"))
{
string blocks;
tileSet.Tiles[tileType].Properties.TryGetValue("Blocks", out blocks);
if (blocks == "true")
_collisionHandler.Insert(_tiles[i, j]);
}
}
}
}
private int GetTileId(TiledMap map, TiledMapTileset tileSet, int id)
{
int firstGid = map.GetTilesetFirstGlobalIdentifier(tileSet);
if ((id >= firstGid) && (id < firstGid + tileSet.TileCount))
return id - firstGid;
return -1;
}
(I am not in a position to upload my working project at this stage.)
Once your project is working, do you want to upload it then?
Because itās too difficult for me (iām both a C# and monogame beginner)
Lisa
Hi
Iām also interested to see the project.
If you want, you can put it on github?