I’m trying to get my game to search for files that are not .xnb and have not gone through the content pipeline. All of these files will be contained in a folder called ‘songs’.
At first, I’m only printing their full paths to the debug console as proof that the search has worked.
string rootPath = "Content/Songs";
var files = Directory.GetFiles(rootPath, "*", SearchOption.AllDirectories);
foreach (string file in files)
{
System.Diagnostics.Debug.WriteLine(Path.GetFullPath(file));
}
The search function only returns a file if the file property ‘Copy to Output Directory’ has been set to ‘copy always’ or copy if newer’. However, the files default to ‘Do not copy’ and so the search never returns the files.
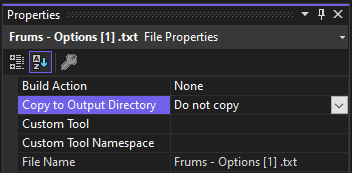
How can I search for the files without manually changing their ‘Copy to Output Directory’ property to ‘Copy if newer’ or putting the files through the content pipeline? Should I move the ‘Songs’ directory somewhere else or use another search function?
It’s important they remain as normal files so that, down the line, new songs can be added freely without going through the pipeline. Files are added to a list later using Uri
.
I’m quite new to coding so any criticism at all is highly appreciated :))
By default, any non-.cs
files, when you add them to your project directory, will not be copied over to your build /bin
directory. This is the default behavior of Visual Studio, which is why you have to manually change the Copy to Output Directory property of that file each time.
But there’s hope 
When you change the Copy to Output Directory, what this is actually doing is editing the .csproj
file for your project to include something like this
<ItemGroup>
<None Update="song.mp3">
<CopyToOutputDirectory>Always</CopyToOutputDirectory>
</None>
</ItemGroup>
It will create an entry for each file you tell it to copy to the output directory. Thankfully you can use a wildcard as the filename to capture ALL mp3 (or ogg or whatever file type you use for songs).
So, for instance, if all of your Songs were in a folder called Songs
in your project directory, you can update your .csproj
to include the following and it will always copy over every .mp3
file in that directory to your build output
<ItemGroup>
<None Update="./Songs/*.mp3" CopyToOutputDirectory="PreserveNewest" />
</ItemGroup>
Doing this, if they were in a folder called Songs in your project directory, this would copy over all .mp3 files in the Songs directory to a Songs directory in your build directory.
2 Likes
I also edited your title from Dictionary.GetFiles
to Directory.GetFiles
1 Like
Thank you so much! This solved my problem perfectly 
2 Likes