I was wondering why there was no overload of the spritebatch.draw method that took float values instead of int values for the destination rectangle (rectangle accepts ints only), especially since the ints are converted to floats afterwards (a bit of a waste, isn’t it?)
I thought it was just something that XNA did and somehow nobody bothered changing it. I think i’ve seen a request to implement float rectangles for spritebatch somewhere.
Anyways, I copied the thing and changed two lines basically to make it accept floats. Instead of rectangle the input is Vector4 now, but the same rules apply (x y width height)
public void Draw(Texture2D texture,
Vector4 destinationRectangle,
Rectangle? sourceRectangle,
Color color,
float rotation,
Vector2 origin,
SpriteEffects effects,
float layerDepth)
So then I was eager to test out my creation and have two blocks slowly move to the right.
Because rectangle accepts ints only the movement is jumpy (from pixel to pixel), and I hoped to fix that with this new input.
To my surprise it didn’t fix anything, the jump just occured on different frames.
I was baffled at first, since I expected smooth interpolation now, since any value of a pixel could now be covered instead of just 1/0 but I forgot the rasterizer.
So the fix was fairly simple:
graphics.PreferMultiSampling = true;
Top: using Vector4() as destination
Below: using Rectangle() as destination
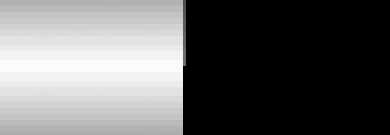
The gif does not give the whole thing justice, since even the fade appears to be unsmooth because the .gif program does not capture at perfect intervals. With faster movement even it is remarkably more calm and less jerky.
TLDR: Making spritebatch more useful just amounts to changing Rectangle to Vector4 in the monogame source.
The question is whether or not creating a dozen overloads for each draw() version with Vector4 is worth it, for me it’s not.