I’m not yet properly familiar with using external classes.
I added a class (test.cs) to my project.
using System;
using System.Collections.Generic;
using System.Text;
using Microsoft.Xna.Framework;
namespace Project1
{
class test
{
protected Matrix view;
protected Matrix proj;
public Matrix View
{
get
{
return view;
}
}
public Matrix Projection
{
get
{
return proj;
}
}
}
}
Now I want to use it in the main class.
I am using the XNA Game Studio 4.0 Programing, to try and understand things.
It says…
Add a new variable to your Game class to hold the camera [:CameraComponent.cs]
CameraComponent camera;
Then, in your game’s Initialize overload, add this component to your game’s component list:
Components.Add(camera = new CameraComponent(this));
This is a Component class, I know, but I thought it would not make a difference. It does, but regardless, I don’t get either to work.
The Component class looks like this…
using System;
using System.Collections.Generic;
using System.ComponentModel;
using System.Diagnostics;
using System.Text;
namespace Project1
{
public partial class tester : Component
{
public tester()
{
InitializeComponent();
}
public tester(IContainer container)
{
container.Add(this);
InitializeComponent();
}
}
}
With this Components.Add(testing = new tester(this));
, I get the error message:

With this Components.Add(tests = new test(this));
, I get the error message:

I’m a baby when it comes to using external classes.
Help would be greatly appreciated.
Your class needs to inherit from either GameComponent or DrawableGameComponent and have a constructor that takes a Game object
public class Test : DrawableGameComponent
{
public Test(Game game) : base(game)
{
}
}
Hope this helps,
pherbeas
Thanks. I did try that, based on an example I used, but I know something is missing, because I get errors.
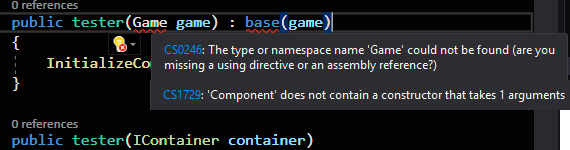
Edit
@pherbeas I just created a class using this code, and there were no errors. Not sure why the other had.
Then I used …
InputManager inM;
Components.Add(inM = new InputManager(this));
there were no errors.
I wish I knew why I got errors on the others, but maybe I will figure it out later. Thanks. 
The reason for the error is a missing using statement.
using statements are placed at the top of a code file, for example:
using System;
or in this case
using Microsoft.Xna.Framework;
If you click on, in this instance, Game, and press Alt + Enter Visual Studio will popup a list of things you can do. One should reference the using you need to add. Click and it will add it for you.
Hope this helps shed some light…
pherbeas
Tester class must inherit from either GameComponent or DrawableGameComponent
public class Tester ---> : GameComponent <---
{
...
}
or
public class Tester ---> : DrawableGameComponent <---
{
...
}
Okay, thanks for your help. 
What do you think of this method?
It worked fine for me.
Do you think this is a very efficient method. Or do you think there is a more preferable way? Just wondering about best practice.
Yeah, I think I watched that series when I first started about a year and a half ago.
I have also watched GamesFromScratch, his videos gave a really good primer on the basics of MonoGame / XNA.
Some other good resources are RB Whitaker, Shawn Hargreaves and github.com
I have lots of throw away projects trying different approaches and figuring out what works through experimenting and reading, blogs and other developer’s code. I am in no way an expert and I still have a lot to learn.
And most importantly, have fun!
pherbeas
1 Like
Great! I think I will use CodingMadeEasy
I like how he takes the time to explain stuff. That’s my kind of tutorials. 