So it looks like furthest triangles render instead of closest ones. In my code below I use _model.Draw() but I already tried drawing model per mash and even using GraphicsDevice.DrawIndexedPrimitives(). Model shows correctly on blender and online viewers. Currently I’m using .dae model format but I had to import it using FBX importer because of another issue. FBX format has the same issue. I also tried using free sword model from store and It had same issue. Found guy with same problem with XNA, post made 11 years ago. He was adviced to enable depthBuffer but this solution did not help me.
namespace VideoGame
{
public class Game1 : Game
{
private GraphicsDeviceManager _graphics;
private SpriteBatch _spriteBatch;
public World _world;
public Game1()
{
_graphics = new GraphicsDeviceManager(this);
_graphics.PreferHalfPixelOffset = true;
Content.RootDirectory = "Content";
IsMouseVisible = true;
}
protected override void Initialize()
{
GraphicsDevice.SetRenderTarget(new RenderTarget2D(
GraphicsDevice, 1920, 1080,
false, SurfaceFormat.Color,
DepthFormat.Depth24, 6,
RenderTargetUsage.PlatformContents));
_graphics.PreferredBackBufferWidth = 1903;
_graphics.PreferredBackBufferHeight = 969;
_graphics.PreferMultiSampling = true;
_graphics.SynchronizeWithVerticalRetrace = true;
this.IsFixedTimeStep = false;
this.TargetElapsedTime = TimeSpan.FromSeconds(1 / 60f);
_graphics.ApplyChanges();
Window.AllowUserResizing = true;
Window.Title = "Game";
DepthStencilState depthStencilState; depthStencilState = new DepthStencilState();
depthStencilState.DepthBufferFunction = CompareFunction.LessEqual;
depthStencilState.DepthBufferWriteEnable = true;
depthStencilState.DepthBufferEnable = true;
GraphicsDevice.DepthStencilState = depthStencilState;
base.Initialize();
}
protected override void LoadContent()
{
_spriteBatch = new SpriteBatch(GraphicsDevice);
_spriteBatch.Begin();
_world =
new World(
new GameClient(Window.ClientBounds, LevelConstructors.CreateKeyBoardControls(), GameClient.GameLanguage.English),
new SpriteDrawer(GraphicsDevice, Content
));
_world.AddLevel(new Level("Level1", LevelConstructors.LoadLevel1));
_world.AddLevel(new Level("Remote Room", LevelConstructors.LoadRemoteRoom));
_world.AddLevel(new Level("Menu", LevelConstructors.LoadMenu));
_world.LoadRootLevel("Level1", Content);
_spriteBatch.End();
projectionMatrix = Matrix.CreatePerspectiveFieldOfView(MathHelper.ToRadians(45),
GraphicsDevice.DisplayMode.AspectRatio,
1, 10000);
viewMatrix = Matrix.CreateLookAt(camPosition, camTarget, Vector3.Up);
worldMatrix = Matrix.CreateWorld(camTarget, Vector3.Forward, Vector3.Up);
_model = Content.Load<Model>("sword");
}
Vector3 camTarget = new Vector3(0f, 0f, 0f);
Vector3 camPosition = new Vector3(0f, 0f, -10f);
Matrix projectionMatrix;
Matrix viewMatrix;
Matrix worldMatrix;
Model _model;
protected override void Update(GameTime gameTime)
{
//_world.Update(gameTime.ElapsedGameTime, Window);
if (Keyboard.GetState().IsKeyDown(Keys.Up))
{
worldMatrix *= Matrix.CreateRotationX(MathHelper.ToRadians(1));
}
if (Keyboard.GetState().IsKeyDown(Keys.Down))
{
worldMatrix *= Matrix.CreateRotationX(-1 * MathHelper.ToRadians(1));
}
if (Keyboard.GetState().IsKeyDown(Keys.Left))
{
worldMatrix *= Matrix.CreateRotationY(MathHelper.ToRadians(1));
}
if (Keyboard.GetState().IsKeyDown(Keys.Right))
{
worldMatrix *= Matrix.CreateRotationY(-1 * MathHelper.ToRadians(1));
}
viewMatrix = Matrix.CreateLookAt(camPosition, camTarget, Vector3.Up);
projectionMatrix = Matrix.CreatePerspectiveFieldOfView(MathHelper.ToRadians(45),
GraphicsDevice.DisplayMode.AspectRatio,
1, 10000);
base.Update(gameTime);
}
protected override void Draw(GameTime gameTime)
{
GraphicsDevice.Clear(Color.CornflowerBlue);
_spriteBatch.Begin(samplerState: SamplerState.PointClamp);
if (_world.IsReady)
{
//_world.Display(_spriteBatch);
}
_spriteBatch.End();
_model.Draw(worldMatrix, viewMatrix, projectionMatrix);
base.Draw(gameTime);
}
}
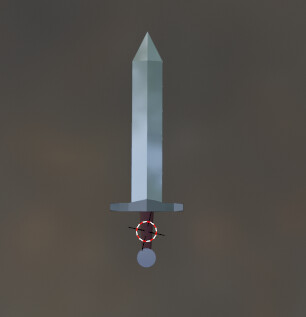
here’s the model Itself
https://drive.google.com/drive/folders/10mmEY2Mw2U8pXMxTMgoeJIHN6T25nd67?usp=sharing