Hey, i wrote a shader that can use the 16 Textures on the GPU.
Question 1:
The problem is i want to use all Slots if a GPU can handle more than the Standard value from Monogame of 16. Is there a way to do this(i already implemented the Monogame library with a custom Drawcall to use them).
Question 2:Also Is there a way to make a sampler array?
sampler Texture : register(s0);
sampler Texture1 : register(s1);
sampler Texture2 : register(s2);
sampler Texture3 : register(s3);
sampler Texture4 : register(s4);
sampler Texture5 : register(s5);
sampler Texture6 : register(s6);
sampler Texture7 : register(s7);
sampler Texture8 : register(s8);
sampler Texture9 : register(s9);
sampler Texture10 : register(s10);
sampler Texture11 : register(s11);
sampler Texture12 : register(s12);
sampler Texture13 : register(s13);
sampler Texture14 : register(s14);
sampler Texture15 : register(s15);
//PixelShader
float4 SpritePixelShader(VertexShaderOutput input) : COLOR0
{
int index = int(input.texCoord.z);
if (input.texCoord.z == 0)
{
return tex2D(Texture, input.texCoord.xy) * input.color;
}
else if(input.texCoord.z == 1)
{
return tex2D(Texture1, input.texCoord.xy) * input.color;
}
etc…
}
I want to do something like this:
uniform sampler Texture[16]
float4 SpritePixelShader(VertexShaderOutput input) : COLOR0
{
int index = int(input.texCoord.z);
return tex2D(Texture[index ], input.texCoord.xy) * input.color;
}
I am using Monogame Desktop.
Maybe a idea where i can look at or a direction would be nice 
Ps: I want to achieve something like this:
Am I right in thinking you want to do multi texture batching? I’d love to see this implemented in monogame, is on my todo list if I get time.
I already implemented it to a cloned nightly build, but i don’t know how to implement the max Texture slot size for each device. 
Oh sorry I should of read your post more carefully. Btw I’d love to have a peak at the full code if its public 
I’m not sure how you’d do it in monogame. But other implementations I have seen dynamically generated a shader with the number of texture slots for the target platform. Then had a global variable that could be manually set if the programmer wanted to override, but would default to the max on the target platform. Generating shaders at runtime must be possible in MG, so your problem really is how to know what this max slot number is. I guess it may be as simple as detecting the platform. Off the top of my head I think modern mobile devices can use up to 16, whereas desktop is more like 32-64?
So you could just make assumptions about the max slots, but of course if you can detect this that would be better still.
The Problem is Monogame Converts Hlsl to glsl shader, and the conversion shows a error(uniform sampler Texture[16]) if i use the Shader with a Array that can be set with Code. 
The rest should be easier, but didn’t it for now, how to apply the Slort size. OpenGL can give you the max textureslot number so that should no problem. 
Nice, that makes life easier 
Did you generate the shaders via the pipeline at runtime? its quite a specific use case so it could be some obscure bug in the conversion, I don’t know this area of MG very well, but if it goes through the pipeline processing (whether runtime or not) this ought to correctly handle conversion to your target platform. Your beyond my knowledge area here though, you need a pipeline whizz!
I create the Shader with the Monogame Content.mgcb
If i recall correctly monogame already generates the shaders procedurally. There are various macros available in the Github repos… but i think the people that made them are not reading here atm.
If you get to the github repo in the Graphics/Effects/Resources folder there are the macros along the compiled ogfx.
[UPDATE: they have been moved in the MonoGame.Framework/Platform/Graphics/Effect/Resources folder now]
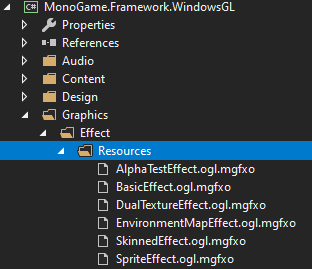
In the latest github there are no Resource Folder? Also im using DesktopGL.
They have been moved to a different folder, under the Platform macro folder:
MonoGame.Framework/Platform/Graphics/Effect/Resources