Hello folks.
I recently came into a problem with drawing pixel-art sprites in my game. I have my game drawn on a RenderTarget2D which is then scaled when drawn to 1920x1080 (the size of my monitor). I was drawing stars on the screen (I’ll put the spritesheet below) but noticed that they only maintained their proper look when having it’s velocity (x or y) a whole number. When changing it from 1 to something like 0.5f, this happens:
This is the spritesheet (scaled only for this post, normally 1x1 pixel ratio):
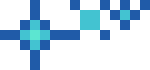
Also, here’s the code where I draw it:
GraphicsDevice.SetRenderTarget(renderTarget);
GraphicsDevice.Clear(Color.CornflowerBlue);
spriteBatch.Begin(samplerState: SamplerState.PointClamp);
stars.Draw(spriteBatch);
spriteBatch.End();
GraphicsDevice.SetRenderTarget(null);
GraphicsDevice.Clear(Color.CornflowerBlue);
spriteBatch.Begin(samplerState: SamplerState.PointClamp);
spriteBatch.Draw(renderTarget, new Rectangle(0, 0, 1920, 1080), Color.White);
spriteBatch.End();
If anyone can help me get to the bottom of this that would be great. Also, I don’t know if this is relevant but I’m scaling the RenderTarget2D using the Rectangle overload, not the Vector2 overload.